//DataFlow 3 to 8 Decoder
module decoder_3to8_dataflow(y,x);
output reg [7:0] y;
input [2:0] x;
always @ (x)
case (x)
3'b000:y=8'b00000001;
3'b001:y=8'b00000010;
3'b010:y=8'b00000100;
3'b011:y=8'b00001000;
3'b100:y=8'b00010000;
3'b101:y=8'b00100000;
3'b110:y=8'b01000000;
3'b111:y=8'b10000000;
endcase
endmodule
Engineering Experiments
NAV BAr
Wednesday, March 8, 2017
2 to 1 MUX
module mul2t1_beha(m,x,y,s);
reg m ;
output [1:0]m;
input [1:0] x,y;
input s;
always @ (x or y or s)
begin
if (s == 0)
m=y;
else m=x;
end
endmodule
//Gate Level 2to1 MUX
////////////////////////////////////////
module mux_2(m,x,s,y);
output [1:0] m;
input [1:0] x, y;
input s;
wire c,d,e,f;
and i(c, x[0], ~s);
and j(d, s, y[0]);
or o(m[0],c,d);
and k(e, x[1], ~s);
and l(f, s ,y[1]) ;
or p( m[1], e,f);
endmodule
Sequence Detector
//Sequence Detector With RTL schematics
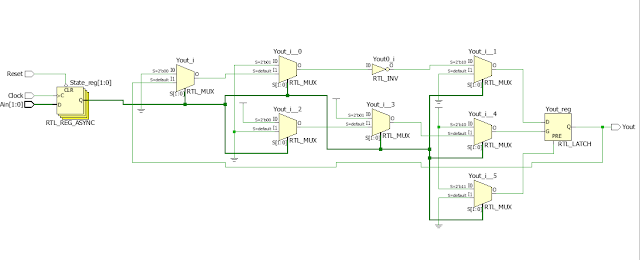
module Variety_sequence_detect(Ain,Yout,Clock,Reset);
input Clock,Reset;
input [1:0] Ain;
output reg Yout;
reg [1:0] State;
parameter S0=2'b00,
S1=2'b01,
S2=2'b10, //toggle state
S3=2'b11; //Led on
always @(posedge Clock,posedge Reset)
begin
if (!Reset)
State<= S0;
else case(Ain)
S0: if (Ain==0 ) State<=S0; else if(Ain==1) State<=S1; else if(Ain==2) State<=S2; else if(Ain==3 ) State<=S3;
S1: if (Ain==1 ) State<=S1; else if(Ain==2) State<=S2; else if(Ain==3) State<=S3; else if(Ain==00 ) State<=S0;
S2: if (Ain==2 ) State<=S2; else if(Ain==1) State<=S1; else if(Ain==3) State<=S3; else if(Ain==0 ) State<=S0;
S3: if (Ain==3 ) State<=S3; else if(Ain==1) State<=S1; else if(Ain==2) State<=S2; else if(Ain==0 ) State<=S0;
endcase
end
always @(State)
begin
if (State==S0) Yout=1'b0;
if (State==S1) Yout=1'b0;
if (State==S2) Yout=~Yout;
if (State==S3) Yout=1;
end
endmodule
module Variety_sequence_detect(Ain,Yout,Clock,Reset);
input Clock,Reset;
input [1:0] Ain;
output reg Yout;
reg [1:0] State;
parameter S0=2'b00,
S1=2'b01,
S2=2'b10, //toggle state
S3=2'b11; //Led on
always @(posedge Clock,posedge Reset)
begin
if (!Reset)
State<= S0;
else case(Ain)
S0: if (Ain==0 ) State<=S0; else if(Ain==1) State<=S1; else if(Ain==2) State<=S2; else if(Ain==3 ) State<=S3;
S1: if (Ain==1 ) State<=S1; else if(Ain==2) State<=S2; else if(Ain==3) State<=S3; else if(Ain==00 ) State<=S0;
S2: if (Ain==2 ) State<=S2; else if(Ain==1) State<=S1; else if(Ain==3) State<=S3; else if(Ain==0 ) State<=S0;
S3: if (Ain==3 ) State<=S3; else if(Ain==1) State<=S1; else if(Ain==2) State<=S2; else if(Ain==0 ) State<=S0;
endcase
always @(State)
begin
if (State==S0) Yout=1'b0;
if (State==S1) Yout=1'b0;
if (State==S2) Yout=~Yout;
if (State==S3) Yout=1;
end
endmodule
Divisible by 3
module divisible_of_three(Ain,Clock,Reset,Yout,Count);
input Ain, Clock, Reset;
output reg Yout;
output reg[3:0] Count;
reg [2:0] State;
parameter S0 = 3'b000,
S1 = 3'b001,
S2 = 3'b010,
S3= 3'b011;
// State Logic
always @ ( posedge Clock or posedge Reset )
begin
if (Reset)
begin
State <= S0;
Count=0;
end
else case (State)
S0: if (Ain==1)
begin
State= S1;
Count<= Count+1;
end
else State<=S0;
S1: if (Ain==1)
begin
State= S2;
Count<= Count+1;
end
else State<= S1;
S2: if (Ain==1)
begin
State= S3;
Count<= Count+1;
end
else State<= S2;
S3: if (Ain==1)
begin
State= S1;
Count<= Count+1;
end
else State<= S3;
default: State <= S0;
endcase
end
// Output Logic
always @ (State)
begin
if (State== S0) Yout =!(1*!Reset);
if (State== S1) Yout = 1'b0;
if (State== S2) Yout = 1'b0;
if (State== S3) Yout = 1'b1;
end
endmodule
input Ain, Clock, Reset;
output reg Yout;
output reg[3:0] Count;
reg [2:0] State;
parameter S0 = 3'b000,
S1 = 3'b001,
S2 = 3'b010,
S3= 3'b011;
// State Logic
always @ ( posedge Clock or posedge Reset )
begin
if (Reset)
begin
State <= S0;
Count=0;
end
else case (State)
S0: if (Ain==1)
begin
State= S1;
Count<= Count+1;
end
else State<=S0;
S1: if (Ain==1)
begin
State= S2;
Count<= Count+1;
end
else State<= S1;
S2: if (Ain==1)
begin
State= S3;
Count<= Count+1;
end
else State<= S2;
S3: if (Ain==1)
begin
State= S1;
Count<= Count+1;
end
else State<= S3;
default: State <= S0;
endcase
end
// Output Logic
always @ (State)
begin
if (State== S0) Yout =!(1*!Reset);
if (State== S1) Yout = 1'b0;
if (State== S2) Yout = 1'b0;
if (State== S3) Yout = 1'b1;
end
endmodule
8 to 3 Encoder
//Design are synthesizable with bit stream
module enc0der8to3(v,en_in_n,y,gs,en_out);
input [7:0] v;
input en_in_n;
output reg [2:0]y;
output reg gs;
output reg en_out;
always @(v,en_in_n)
begin
if(en_in_n == 1'b1)
begin
y = 3'b111;
en_out =1'b 1;
gs= 1'b1;
end
else if(v==8'b11111111 && en_in_n== 1'b0)
begin
y= 3'b111;
gs=1'b1;
en_out=1'b0;
end
else if(v==8'b0 && en_in_n== 1'b0)
begin
y= 3'b000;
gs=1'b0;
en_out=1'b1;
end
else if(v==8'b01 && en_in_n== 1'b0)
begin
y= 3'b001;
gs=1'b0;
en_out=1'b1;
end
else if(v==8'b011 && en_in_n== 1'b0)
begin
y= 3'b010;
gs=1'b0;
en_out=1'b1;
end
else if(v==8'b0111 && en_in_n== 1'b0)
begin
y= 3'b011;
gs=1'b0;
en_out=1'b1;
end
else if(v==8'b01111 && en_in_n== 1'b0)
begin
y= 3'b100;
gs=1'b0;
en_out=1'b1;
end
else if(v==8'b011111 && en_in_n== 1'b0)
begin
y= 3'b101;
gs=1'b0;
en_out=1'b1;
end
else if(v==8'b0111111 && en_in_n== 1'b0)
begin
y= 3'b110;
gs=1'b0;
en_out=1'b1;
end
else if (v==8'b01111111 && en_in_n== 1'b0)
begin
y= 3'b111;
gs=1'b0;
en_out=1'b1;
end
else
begin
y= 3'b111;
gs = 1'b1;
en_out=1'b1;
end
end
endmodule
module enc0der8to3(v,en_in_n,y,gs,en_out);
input [7:0] v;
input en_in_n;
output reg [2:0]y;
output reg gs;
output reg en_out;
always @(v,en_in_n)
begin
if(en_in_n == 1'b1)
begin
y = 3'b111;
en_out =1'b 1;
gs= 1'b1;
end
else if(v==8'b11111111 && en_in_n== 1'b0)
begin
y= 3'b111;
gs=1'b1;
en_out=1'b0;
end
else if(v==8'b0 && en_in_n== 1'b0)
begin
y= 3'b000;
gs=1'b0;
en_out=1'b1;
end
else if(v==8'b01 && en_in_n== 1'b0)
begin
y= 3'b001;
gs=1'b0;
en_out=1'b1;
end
else if(v==8'b011 && en_in_n== 1'b0)
begin
y= 3'b010;
gs=1'b0;
en_out=1'b1;
end
else if(v==8'b0111 && en_in_n== 1'b0)
begin
y= 3'b011;
gs=1'b0;
en_out=1'b1;
end
else if(v==8'b01111 && en_in_n== 1'b0)
begin
y= 3'b100;
gs=1'b0;
en_out=1'b1;
end
else if(v==8'b011111 && en_in_n== 1'b0)
begin
y= 3'b101;
gs=1'b0;
en_out=1'b1;
end
else if(v==8'b0111111 && en_in_n== 1'b0)
begin
y= 3'b110;
gs=1'b0;
en_out=1'b1;
end
else if (v==8'b01111111 && en_in_n== 1'b0)
begin
y= 3'b111;
gs=1'b0;
en_out=1'b1;
end
else
begin
y= 3'b111;
gs = 1'b1;
en_out=1'b1;
end
end
endmodule
Tuesday, February 21, 2017
Blinky
/* Blinky for LED's turns on and off with every 100ms ,500ms and 1s using delay function
*/
#include <18F4455.h>
#device ICD=TRUE
#fuses HS,NOLVP,NOWDT,PUT
#use delay(clock=20000000, crystal=20 MHz) // using 20 MHz crystal oscillator
/*Pin Configurations*/
#define FIRST_LED PIN_A0
#define SECOND_LED PIN_A1
#define THIRD_LED PIN_A2
void turn_on(int led)
{
switch (led) {
case 0 :
output_toggle(FIRST_LED);
break;
case 1 :
output_toggle(SECOND_LED);
break;
case 2 :
output_toggle(THIRD_LED);
break;
}
}
void main()
{
int count=1;
while (TRUE){
turn_on(0);
if (count ==5) {
turn_on(0);
turn_on(1);
}
else if (count ==10){
turn_on(0);
turn_on(1);
turn_on(2);
count =1;
}
count=count+1;
delay_ms(100);
}
}
*/
#include <18F4455.h>
#device ICD=TRUE
#fuses HS,NOLVP,NOWDT,PUT
#use delay(clock=20000000, crystal=20 MHz) // using 20 MHz crystal oscillator
/*Pin Configurations*/
#define FIRST_LED PIN_A0
#define SECOND_LED PIN_A1
#define THIRD_LED PIN_A2
void turn_on(int led)
{
switch (led) {
case 0 :
output_toggle(FIRST_LED);
break;
case 1 :
output_toggle(SECOND_LED);
break;
case 2 :
output_toggle(THIRD_LED);
break;
}
}
void main()
{
int count=1;
while (TRUE){
turn_on(0);
if (count ==5) {
turn_on(0);
turn_on(1);
}
else if (count ==10){
turn_on(0);
turn_on(1);
turn_on(2);
count =1;
}
count=count+1;
delay_ms(100);
}
}
Subscribe to:
Posts (Atom)